Getting Started with Python and JSON API Applications: Get Current LikeCoin Price Using Python
In the following example, we get the API URL, test the API, format the JSON output, download the data, and convert the data to text output. Step by step to implement a small program that reads network data in Python.
The final code is as follows:
import requests url = "https://api.coingecko.com/api/v3/simple/price?ids=osmosis,likecoin&vs_currencies=usd" res = requests.get(url) data = res.json() like_usd = data["likecoin"]["usd"] osmo_usd = data["osmosis"]["usd"] result = f"Current LIKE/USD is {like_usd}, OSMO/USD is {osmo_usd}." print(result)
Learn to use CoinGecko API service to query currency prices
For currency price query, we can use CoinGecko to easily obtain API data in JSON format through Python and third-party requests suite.
Faced with any new understanding of API services, we can first understand, such as how to authenticate login, price, etc. General API services require users to register an account and set a limit on the number of requests.
https://www.coingecko.com/en/api/pricing
CoinGecko has it too. The free version does not require registration, but under fair use, it should be no more than 50 requests per minute, and you must also explain that you use their service.
Secondly, when we encounter a new API library, we can also read their documentation first to see if we can get what we want and use it for ourselves.
https://www.coingecko.com/en/api/documentation
From the documentation, we can see how different data are provided by CoinGecko's API. It can be seen that we can use this API library to create more applications in the future. But the downside of having too many features is that it's harder to find the API location we need. Fortunately, CoinGecko put the most basic RFQ API at the top of the list. It is /simple/price
.
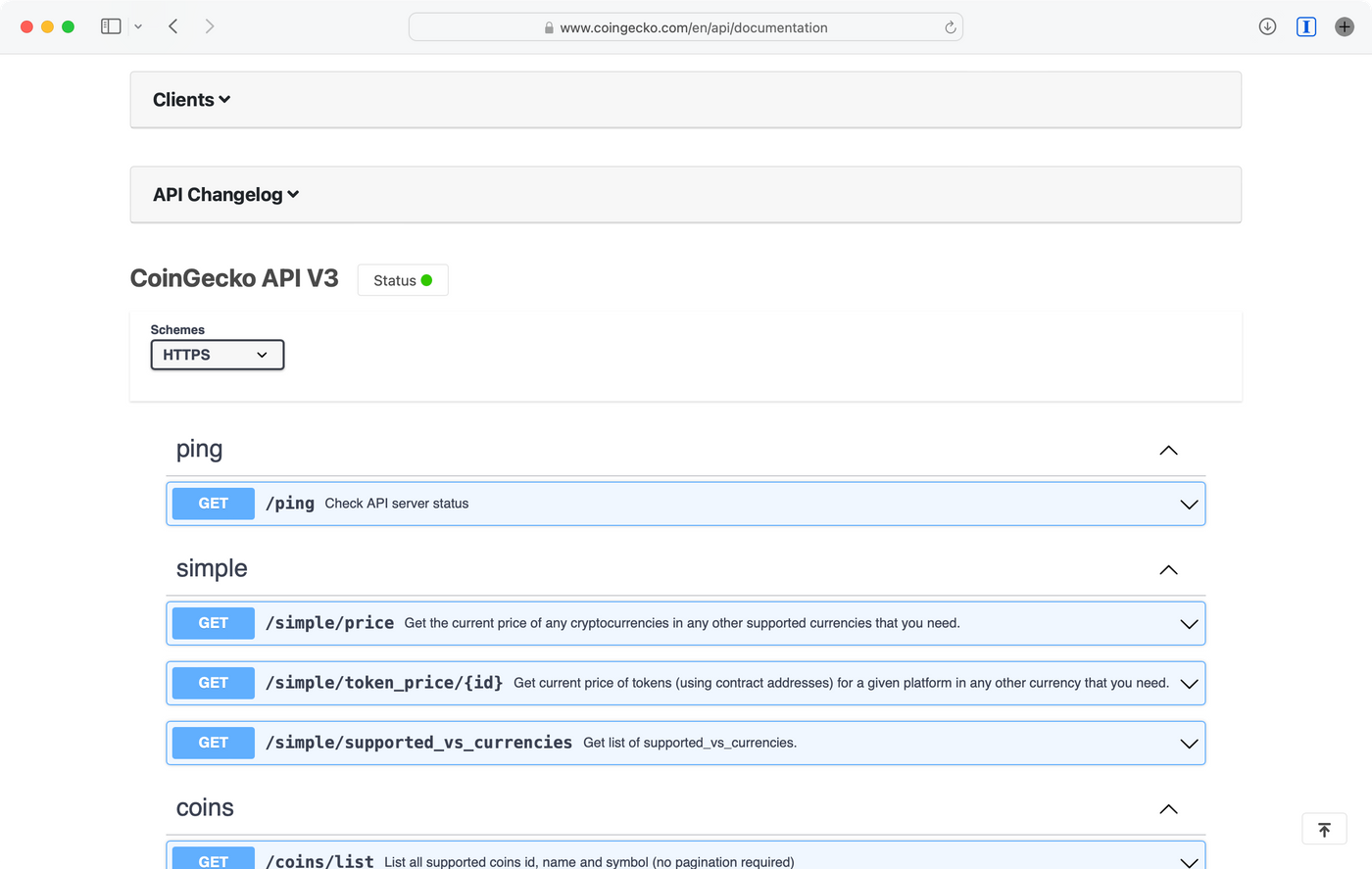
CoinGecko's documentation can try the API directly. After selecting the /simple/price
API, click Try it out, and fill in the two necessary parameters as instructed: ids
and vs_currencies
. With the s
at the end of the parameter name and the "comma separated" of the description text, we know that both parameters can be given to multiple currencies.
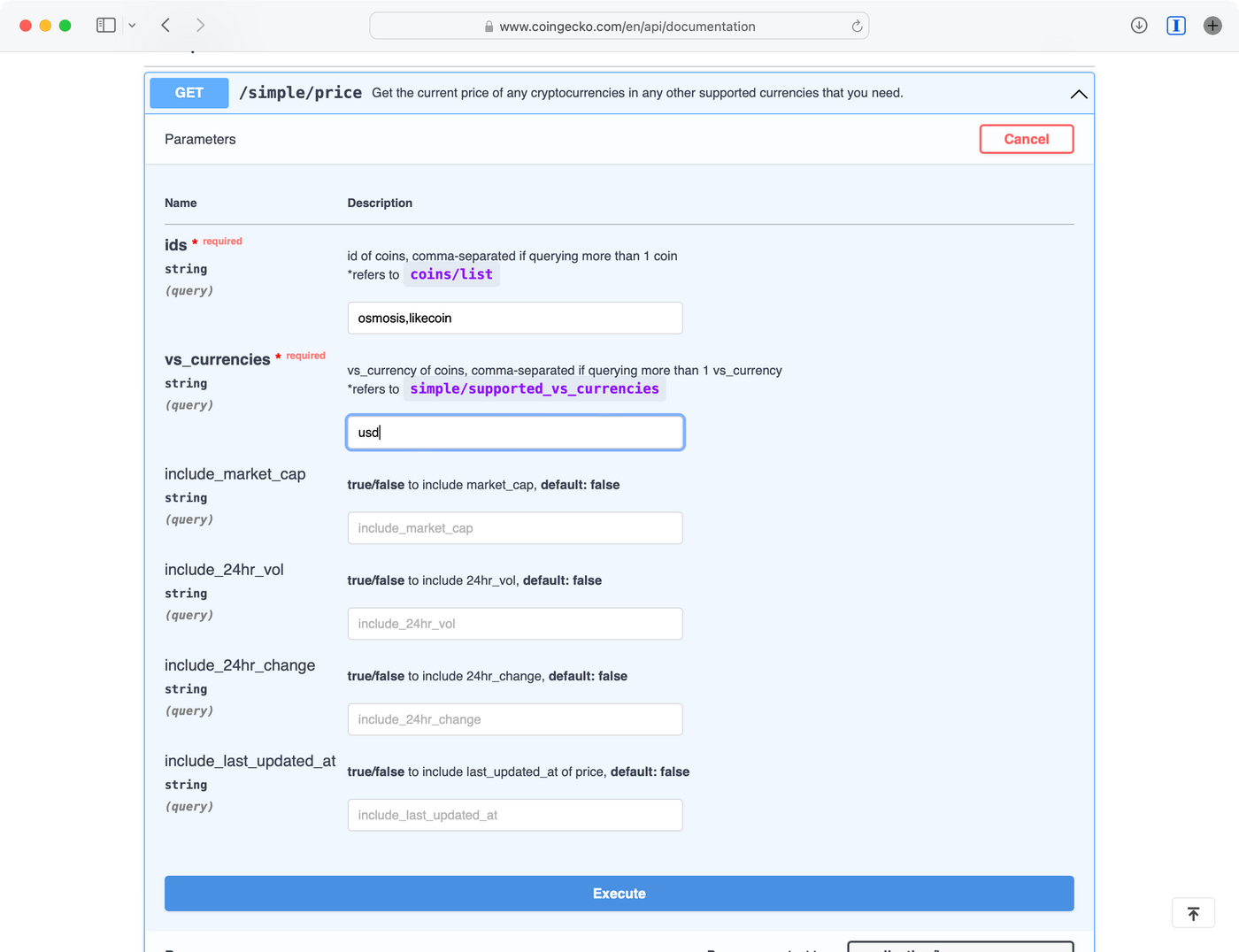
In other words, the cryptocurrency id
of CoinGecko is not short, but long, that is, likecoin
and osmosis
.
After filling in the parameters, you can execute and get the API result of the trial operation: it is a data structure with an intuitive structure.
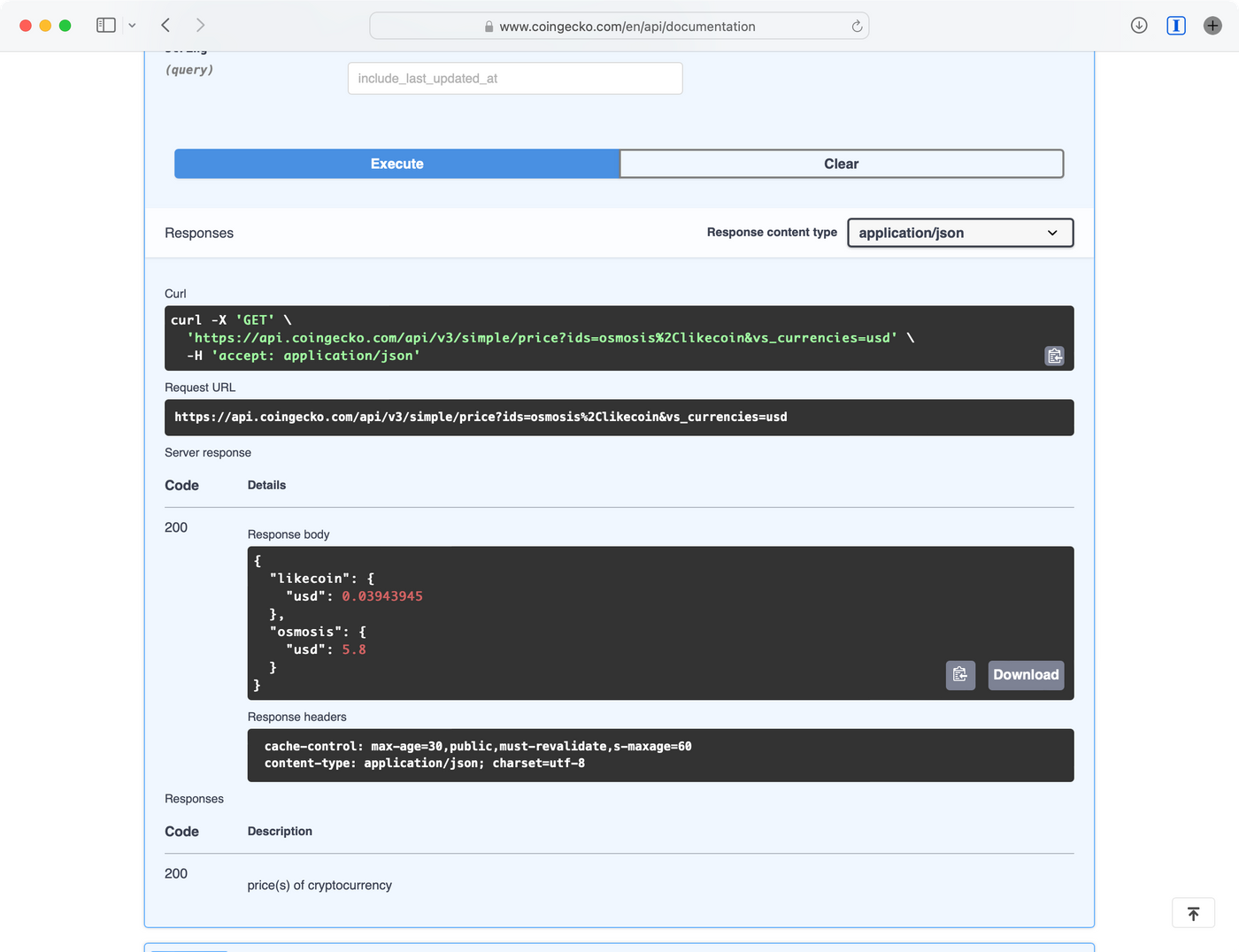
Through testing, we can conclude that the API End Point URL of this example is:
https://api.coingecko.com/api/v3/simple/price?ids=osmosis,likecoin&vs_currencies=usd
When we take the JSON API URL and want to preview the data, we usually find some JSON Formatter to make reading JSON more convenient. But in fact, the Firefox browser also has a built-in useful JSON formatted browsing tool. In addition to being easy to read, you can also search and filter when the return value is too large.
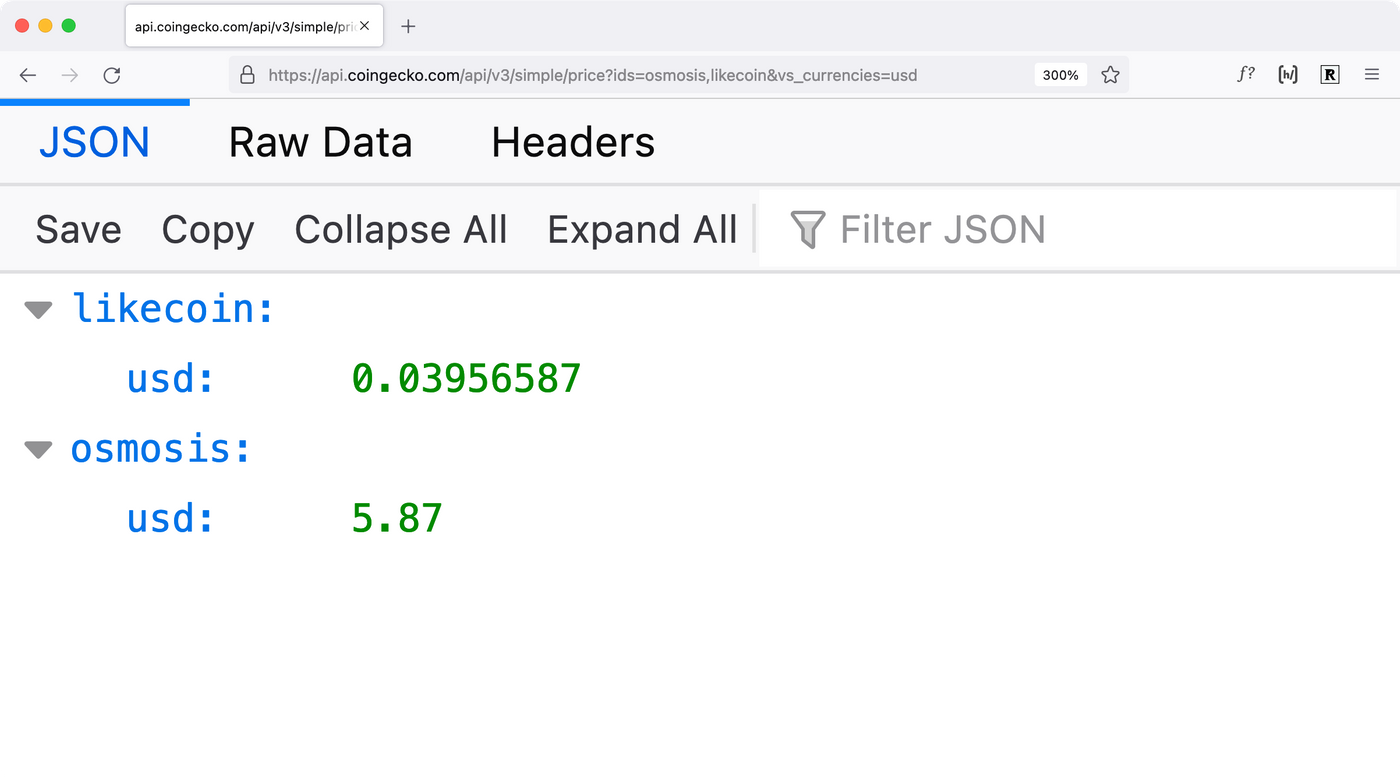
Python development environment
Next comes the Python part.
In learning, I like to use Anaconda with Jupyter Notebook , because I can open another test code at any time without affecting the structure of the code I am writing. (Of course, you can also follow with other Python development environments)
We will use the third-party library of requests
this time. We import requests
. If there is an error that requests cannot be found, it is not installed. It can be installed through the pip install requests` command.
Next, we can call requests.get(url)
. To access resources from the network, the main operation is either GET or POST. POST is generally used when the form is sent out, while GET is used to obtain resources. For example, enter the URL in the browser URL and press Enter. GET request.
So we are using requests.get
request. After the request is made, we record and use the response that comes back. First, print the response and take a look. If you see that the status is 200, the return is successful. Except for 200, there are errors in the 4-header and 5-header, such as the most common 404 not found.
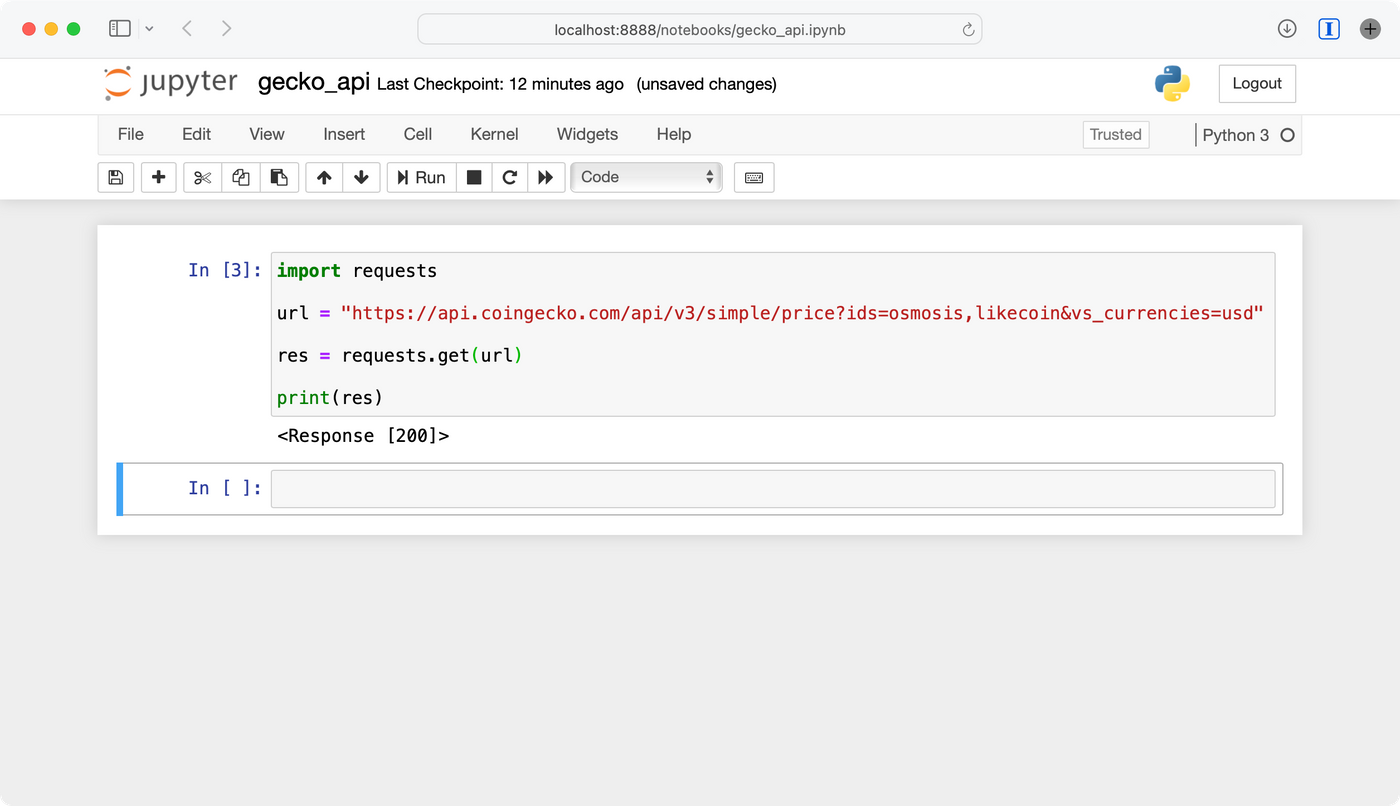
The return status is 200, which means the normal return value. Where is the data value? You can view the returned content through res.text
. Since it is JSON, you can also directly convert the returned data into Python's dict dictionary type through res.json()
. I store the data in the data
variable. At this time, we can obtain data through data["likecoin"]["usd"]
and data["osmosis"]["usd"]
.
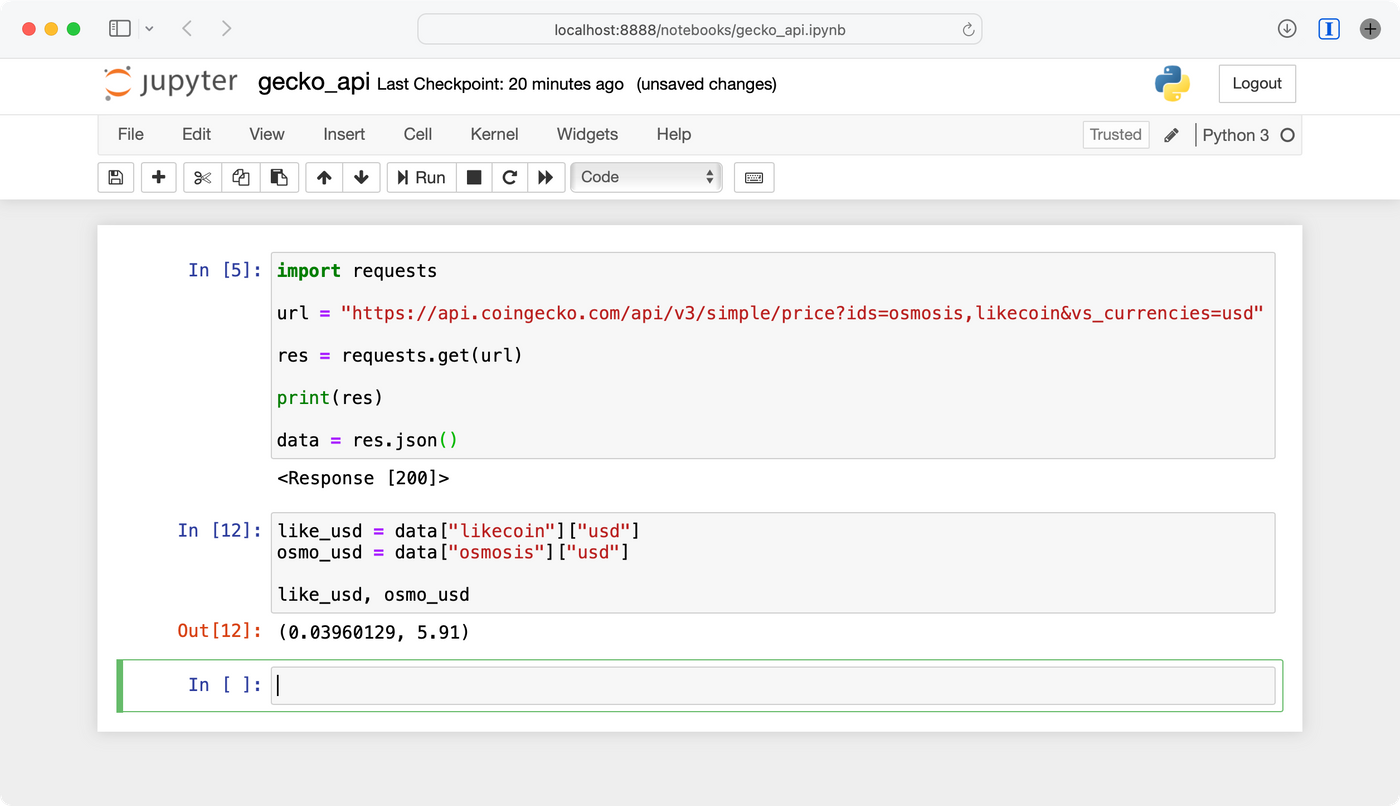
Cooking programming method: materials, processing, serving
After obtaining the value we want, it is like cooking, the material ( data
) is ready, the processing is completed ( like_usd
and osmo_usd
), and the last step is to serve the food, that is, to output the result.
I first try to print the content in one sentence, for example: "Currently LIKE is 0.4 against the US dollar, and OSMO is 5.91 against the US dollar."
After having the output idea, add {}
and the corresponding variable name to the numerical part that needs to be dynamic, and then add f
at the front of the string, and it becomes a dynamic formatted text (f-string).
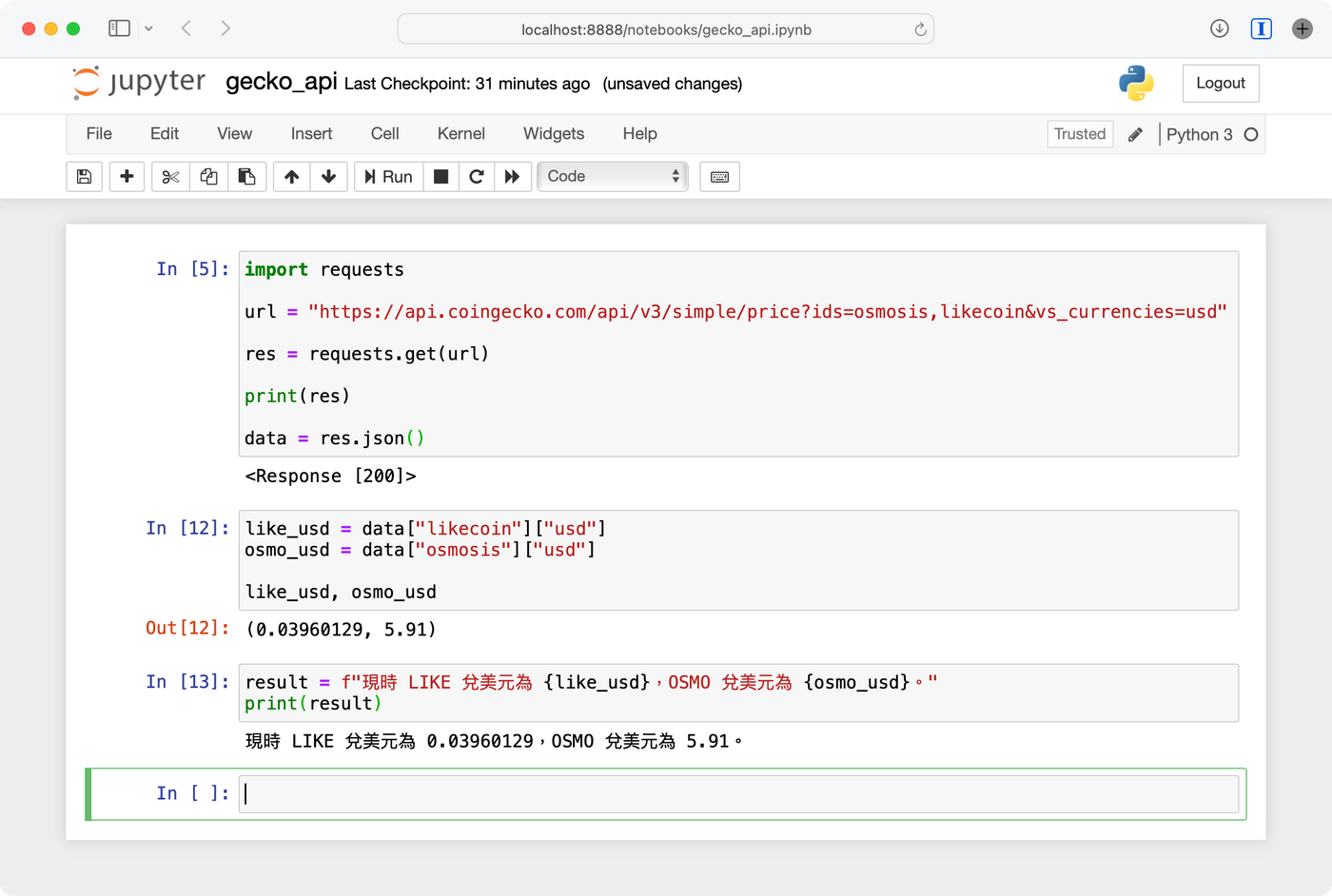
So far, a basic Python request JSON API to get the latest LIKE and OSMO price code is completed.
The final code is as follows:
import requests url = "https://api.coingecko.com/api/v3/simple/price?ids=osmosis,likecoin&vs_currencies=usd" res = requests.get(url) data = res.json() like_usd = data["likecoin"]["usd"] osmo_usd = data["osmosis"]["usd"] result = f"Current LIKE/USD is {like_usd}, OSMO/USD is {osmo_usd}." print(result)
Versions that can be tested online:
https://replit.com/@makzan/Example-CoinGecko-Price-API
— Makzan, 2021-11-24.
My name is Makzan . In addition to my full-time job, I usually hold local competitions and world competitions, or teach on-the-job training in programming and website development. Now he is transforming the face-to-face training content into e-books, online teaching materials, etc. So far, he has written 7 books and 2 video teaching courses.
I occasionally launch #ProgrammingWednesday every Wednesday , introducing Python or different programming techniques, including automated office document processing, and web crawling.
If my article is valuable, please subscribe to sponsor me to continue to create and share.
Subscription Sponsorship: https://liker.land/thomasmak/civic
Like my work? Don't forget to support and clap, let me know that you are with me on the road of creation. Keep this enthusiasm together!
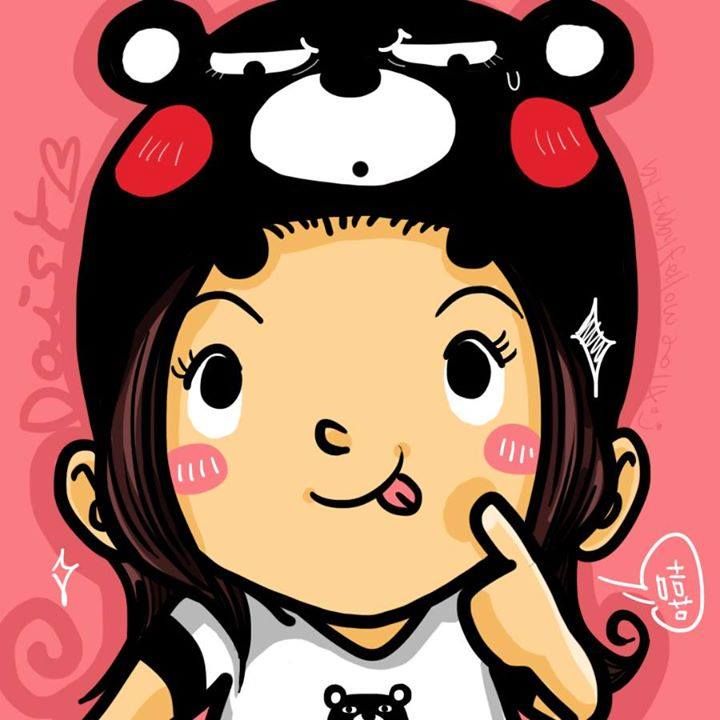
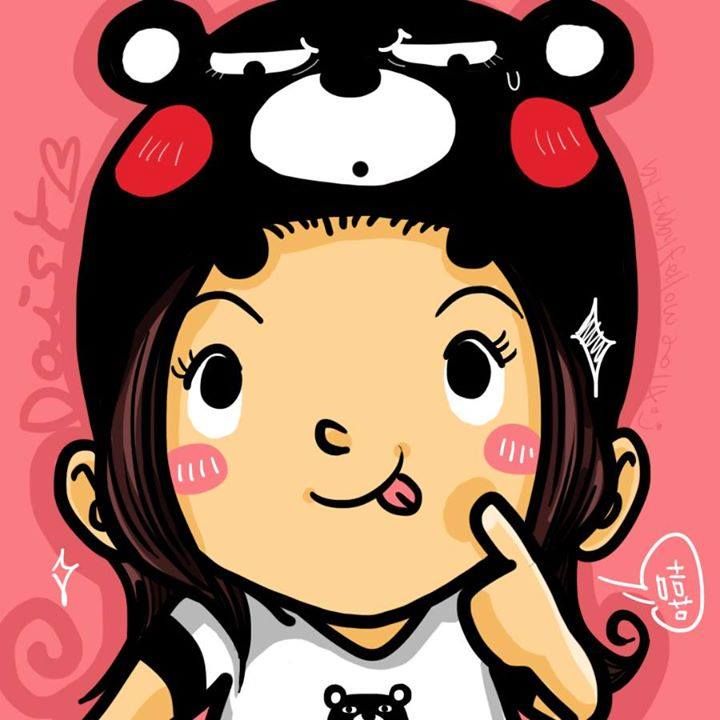
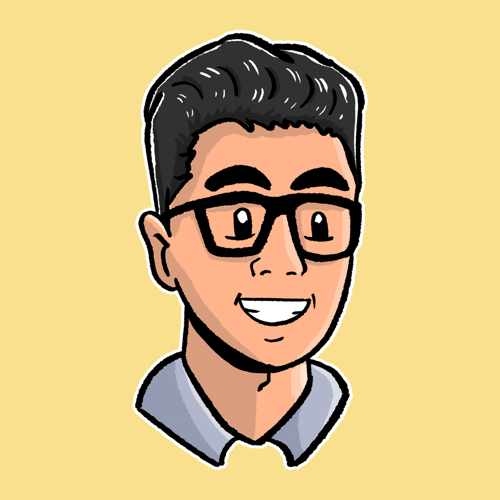

- Author
- More