React.js — Day03
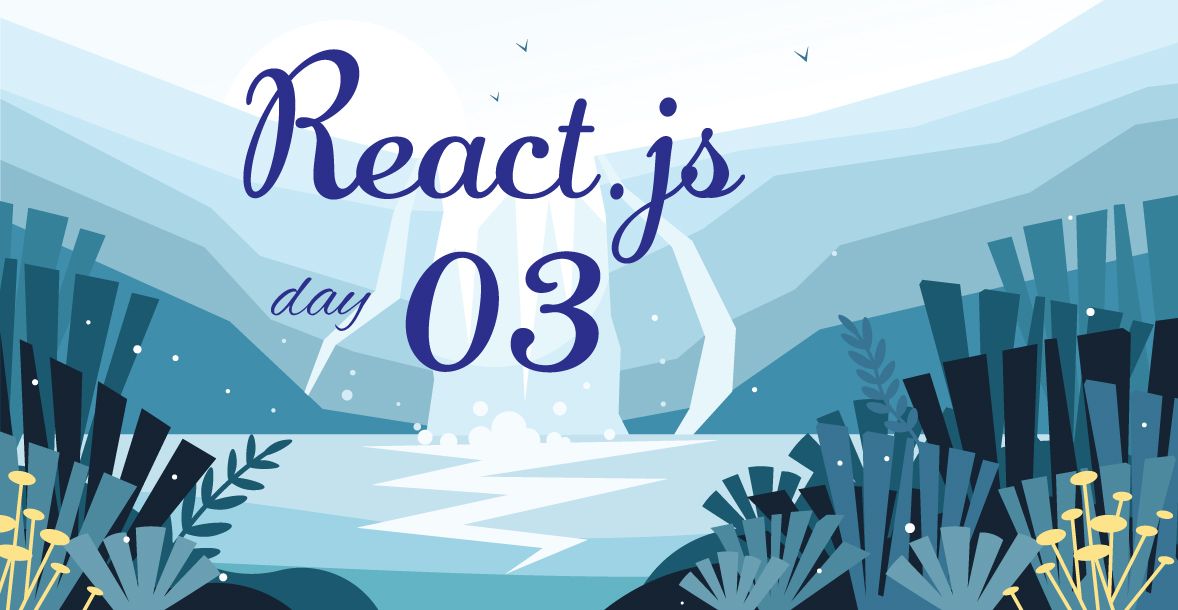
- 寫出像html的語法(syntax)
- Coding方式像是使用視覺化的方式建立並渲染(render)
駝峰式大小寫Camel Case
把首字母的大寫改成小寫,其他字的首字母都是大寫
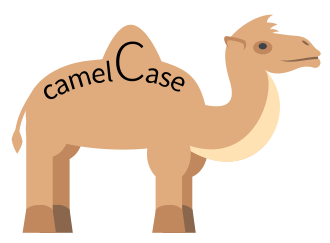
詳細解說 | 在這裡
圖片來自 | Wiki
元件Component
- Components 像是函式(functions) 會回傳( return) HTML元素( elements)
- Components讓你將UI拆開成分別、獨立、可重複利用的程式碼。
注意 |
Component 的字首必須是"大寫字母"
小寫字母會被React 視為原始的DOM標籤。
更多詳情 | 這裡
Rendering element
- 建立 React 應用程式最小的單位是 element。
- React element 是 immutable 的。一旦你建立一個 element,你不能改變它的 children 或是 attribute。
更多解釋 | 好文在此
別將component和element混淆,
概念上來說,component 就像是 JavaScript 的 function,它接收任意的參數(稱之為「props」)並且回傳描述畫面的 React element。
React Render HTML
Render函式,arguments其實就是parameters的意思。
ReactDOM.render()
的函式帶有兩個arguments, HTML 程式碼和一個 HTML element.
函式的目的是去展示在特定HTML element裡,特定的 HTML code 。
在react根目錄中,有個資料夾 “public”,裏頭有Iindex.html
的檔案,你會注意到單一的<div>
在檔案的body裡。這裡就是我們的 React application 會被渲染的地方。
more info | https://www.w3schools.com/react/react_render.asp
React DOM
React DOM比較了 element 和前一個的children ,只讓DOM更新必要的部分,將DOM帶到想要的State。
React events
<button onClick={this.handleClick}>
More examples |
https://www.w3schools.com/react/react_events.asp
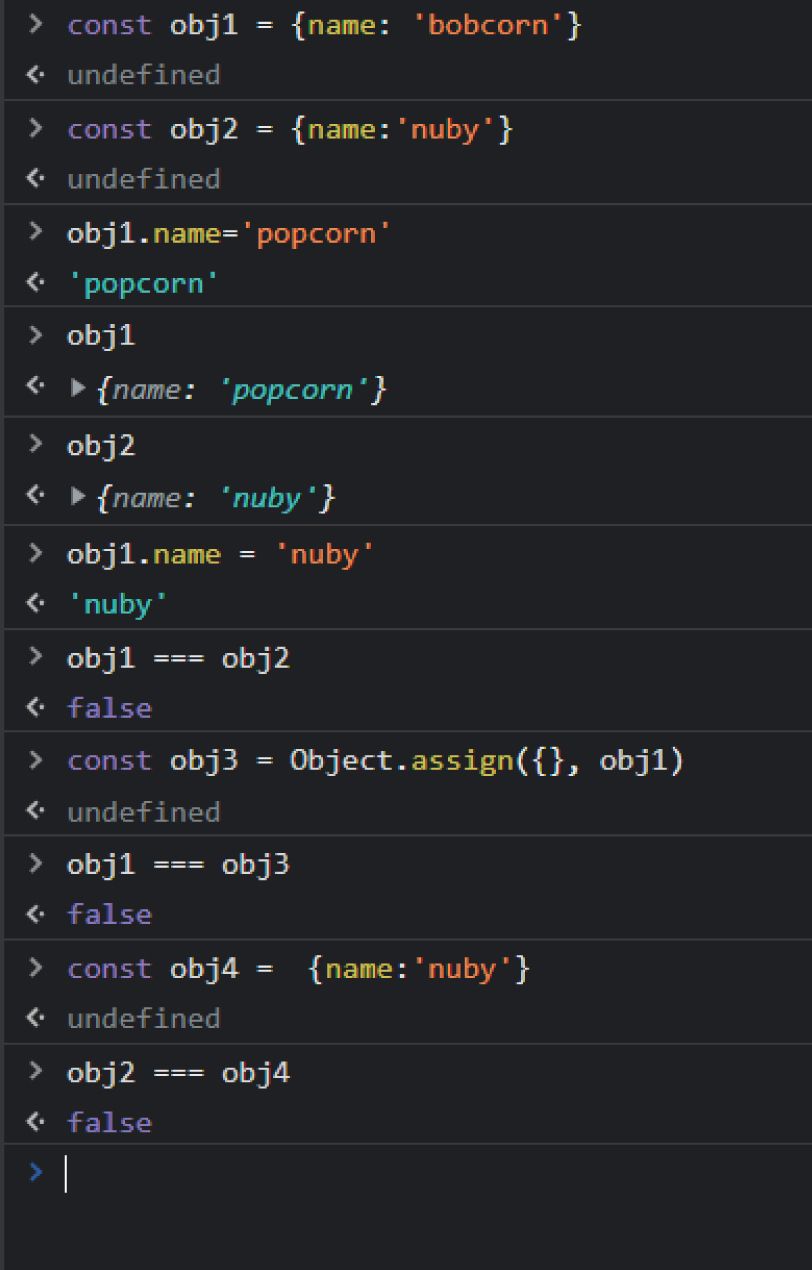
即使他們看起來一樣,但他們在記憶體中的位置不同,也被認作不同的物件。
setState
setState
為非同步
讓UI在React applications被更新的主要方式,就是使用 setState()
函式,此方法會在新的State和前一個state中表現 shallow merge ,此舉會觸發component和後代(descendent)的 re-render。
more info | GeekforGeek
Props
props
被傳到component (和函式 parameters相似) ,
state
在 component 中被處理(類似在函式中宣告的變數)。
Example today
import logo from "./logo.svg"; import { Component } from "react"; import "./App.css"; class App extends Component { constructor() { super(); this.state = { name: { firstName: "Bob", lastName: "Corn" }, company: "BJJ", }; } render() { return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> <p> Hi {this.state.name.firstName} {this.state.name.lastName}, I work at{" "} {this.state.company} </p> <button onClick={() => { this.setState( () => { return { name: { firstName: "You", lastName: "Newbie" } }; }, () => { console.log(this.state); } ); }}> Change Name </button> </header> </div> ); } } export default App;
Mapping & Key Attribute
import { Component } from "react"; import "./App.css"; class App extends Component { constructor() { super(); this.state = { monsters: [ { name: "Goblin" }, { name: "Troll" }, { name: "Orc" }, { name: "Ogre" }, ], }; } render() { return ( <div className="App"> {this.state.monsters.map((monster) => { return <h1>{monster.name}</h1>; })} </div> ); } } export default App;
有個錯誤...

每次在render()中使用 map()
函式 , 他們需要 key attribute。
render() { return ( <div className="App"> {this.state.monsters.map((monster) => { return <h1 key={monster.id}>{monster.name}</h1>; })} </div> ); } }