Javascript in Leetcode 01–03 | 面試問題
IPFS
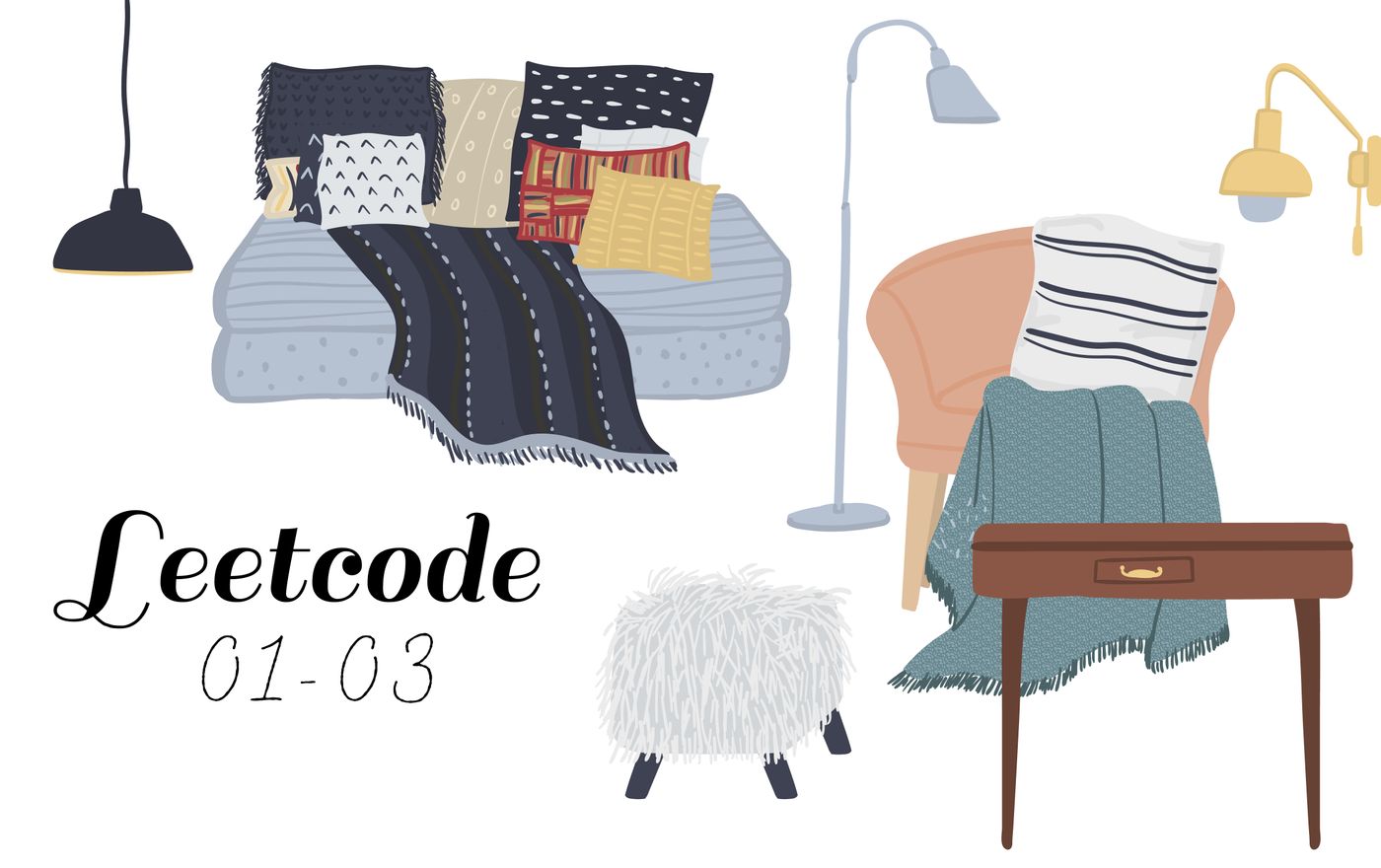
Pheezx Coding
01. Two sum
Input: nums = [2,7,11,15], target = 9 Output: [0,1] Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
solution
function twoSum(nums, target) { const comp = new Map(); const len = nums.length; for(let i=0; i < len; i++){ if(comp[nums[i]] >= 0){ return [comp[nums[i]], i]; } comp[target- nums[i]] = i; } return []; } const nums = [2,7,11,15]; console.log(twoSum(nums,9));
02. Add two numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Input: l1 = [2,4,3], l2 = [5,6,4] Output: [7,0,8] Explanation: 342 + 465 = 807.
Solution
var addTwoNumbers = function(l1, l2) { let carry = 0; let previousNode = new ListNode(); const headNode = previousNode; while(l1 || l2 || carry) { let val1 = 0; let val2=0; if(l1) { val1=l1.val; l1=l1.next; } if(l2) { val2=l2.val; l2=l2.next; } const sum = val1 + val2 + carry; carry = Math.floor(sum / 10); const digit = sum % 10; const currentNode = new ListNode(digit); previousNode.next = currentNode; previousNode = currentNode; } return headNode.next; };
leetcode problems | https://leetcode.com/problems/add-two-numbers/
03. Longest Substring Without Repeating Characters
Input: s = "abcabcbb" Output: 3 Explanation: The answer is "abc", with the length of 3.
Constraints:
0 <= s.length <= 5 * 104
s
consists of English letters, digits, symbols and spaces.
Solution
var lengthOfLongestSubstring = function(s) { let max = 0 let begin = 0 let set = new Set() for(let end = 0; end < s.length; end++){ while(set.has(s[end])){ set.delete(s[begin]) begin = begin + 1 } set.add(s[end]) max = Math.max(max, end - begin + 1) } return max };
喜欢我的作品吗?别忘了给予支持与赞赏,让我知道在创作的路上有你陪伴,一起延续这份热忱!
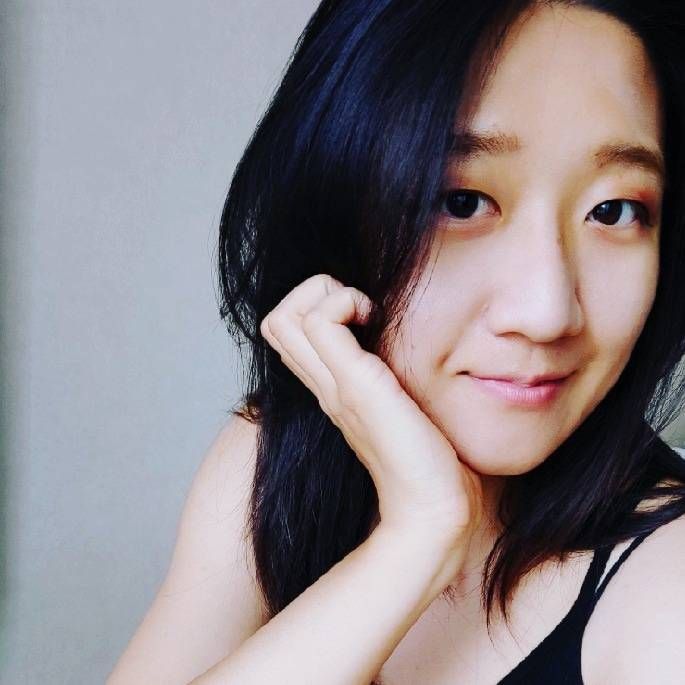
- 来自作者
- 相关推荐