Python Module Concept Analysis
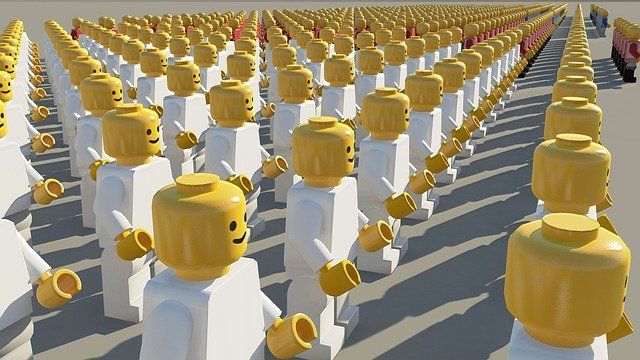
foreword
In the concept of function in Python (Part 1), the concept of function in Python (Part 2) and the concept of function in Python (Part 3) , we are clear through three articles An introduction to the concept of functions in Python. With Functions, we can make the code we write more structured. For example, when we have finished writing the Python code for a function, we can package this code into a Function, so that when we want to use this function, we only need to call this Function , without rewriting the code.
In fact, the power of Python is that there are so many "off the shelf tools" you can use. That is to say, many people have written Python codes for various functions. When we want to use a certain function, we only need to "Import" the corresponding "Module".
Seeing here, there are two words that we have not seen before: Import and Module. In this article, we will introduce what a Module is in Python, how to build a Module, and thoroughly understand the concept of Module in Python.
If your concept of Python Module is quite clear, do you know the difference between Python Module and Python Package ?
What is Module in Python
Module in Python refers to a file containing Python code. For example, if you write "example.ipynb" or "example.py" in Colab, you can treat it as a Module, and the name of this Module is "example".
You might be surprised, is that what Module is all about? So are the Python files we usually write as Modules?
That's right! We can think of a file containing Python code as a Python Module, but the code in a Module usually has one feature: "reusable" .
For example, we will package the functions we often use into many Functions, and then we will put these Functions in a Python file, such as "tool.py". When we want to use these functions in other programs, we can Import this Module, or only one of the Functions in the Import Module. This way, we don't have to rewrite an identical Function.
Create a Python Module
After a little understanding of Modules in Python, let's start building a Module.
First open the Colab environment and name the Colab file "main.ipynb", then in the area of the file list on the left, right-click and "Add File":
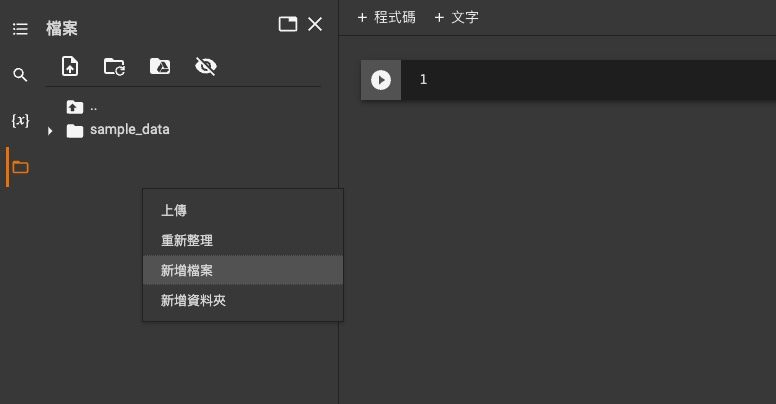
We named the file "tool.py", indicating that the name of this Module is "tool".
Next, open tool.py and type the following code in it:
def sum_mul(a, b, c): return (a+b)*c
We've added a Function to the Module that calculates the sum of two numbers multiplied by a third number.
Introduce Module and use Function in Module
Next, we have to start writing programs in our current Colab file (main.ipynb) and use the Function in the tool Module.
However, in main.ipynb it is not aware of the existence of the tool Module, let alone to use the Function in the tool Module. Therefore, we need to import the tool Module into main.ipynb through the "import" keyword in Python:
import tool
In this way, main.ipynb knows the existence of the tool Module.
Although main.ipynb knows the existence of the tool Module, it does not know what Functions are in the tool Module. Therefore, if you want to use the Function we just defined in the tool Module, you must use the special symbol " . ":
print(tool.sum_mul(1, 2, 3))
4 Ways to Introduce Modules
In fact, there are many ways to introduce Module, which can be mainly divided into the following four, let us understand them one by one.
Simply introduce Module
The first is to simply introduce a Module and access the code in the Module through ".".
import tool print(tool.sum_mul(1, 2, 3))
Introduce Module while giving Module a new name
Through the "as" keyword, give the Module a new name, so that when accessing the Function in the Module later, we don't have to type so many words.
import tool as t print(t.sum_mul(1, 2, 3))
Introduce only certain elements in Module
Sometimes, we don't want to import the entire Module, because we only use one or two elements in the Module. At this point, we can import specific elements in Module through the "from" keyword. In this way, we can use those elements directly in the code behind without going through Module.
from tool import sum_mul print(sum_mul(1, 2, 3))
Import all elements in Module
If there are many elements in a Module (such as many Functions), it will be very laborious to import through the third method. At this time, we can import all the elements in the Module through the "*" symbol. In this way, we can also use those elements directly in the code behind, without going through Module.
from tool import * print(sum_mul(1, 2, 3))
There is a small reminder here, usually we do not recommend to introduce all the elements in the Module, which will easily increase the chance of program errors.
For example, suppose we are now writing our program in main.ipynb, and I define a Function called "sum_mul":
def sum_mul(a, b, c, d): return (a+b+c)*d
Next, I want to use the functions in the tool Module, so I import all the elements in the tool Module:
from tool import *
At this time, what happens when we use the sum_mul Function we just defined?
sum_mul(1, 2, 3, 4) -------------------------------------------------- ------------------------- TypeError Traceback (most recent call last) <ipython-input-14-ceb4a433275d> in <module>() ----> 1 sum_mul(1, 2, 3, 4) TypeError: sum_mul() takes 3 positional arguments but 4 were given
We found that sum_mul( ) cannot be executed correctly, because it accepts 3 parameters, but we pass 4 parameters in!
The reason for this is that when we introduce all the elements in the tool Module, the sum_mul( ) Function in the tool Module "overrides" our original definition of the sum_mul( ) Function.
Therefore, when we introduce a Module, the most recommended approach is to " simply introduce a Module " or " introduce a Module while giving the Module a new name ".
Standard Modules in Python
There are many built-in, pre-built modules in the Python programming language, which we can view in the Python Module Index .
All Modules presented in the Python Module Index are Modules that are automatically downloaded when we install the Python programming language on our own computer. When we want to use these Modules, we just need to use the various import methods mentioned above to import the Module into our program.
For example, there is an "os" Module in Python's Standard Module:
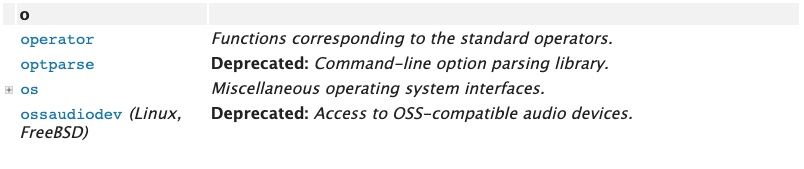
Various operations related to the operating system are defined in the "os" Module. For example, if we want to list all files in a folder, we can use the functions in os Module.
Epilogue
In this article, we introduced the concept of Module in Python, understanding that the so-called Python Module is actually a Python file containing many "reusable" code. We also introduced 4 ways to introduce Python Modules, among which "simply introduce Module" or "Introduce Module and give Module a new name" is the most recommended to avoid errors during program execution.
In the next article , we'll build on your understanding of Python Modules to see why some people write in Python archives:
if __name__ == "__main__":
What about such code?
👣 👣 👣 I like to write articles related to programming and data science. I hope I can explain complex concepts through simple words! If you are also interested, you can visit my other platforms!
👉🏻 DataSci Ocean
👉🏻 YouTube
👉🏻 Instagram
👉🏻 Potato Media
Like my work? Don't forget to support and clap, let me know that you are with me on the road of creation. Keep this enthusiasm together!
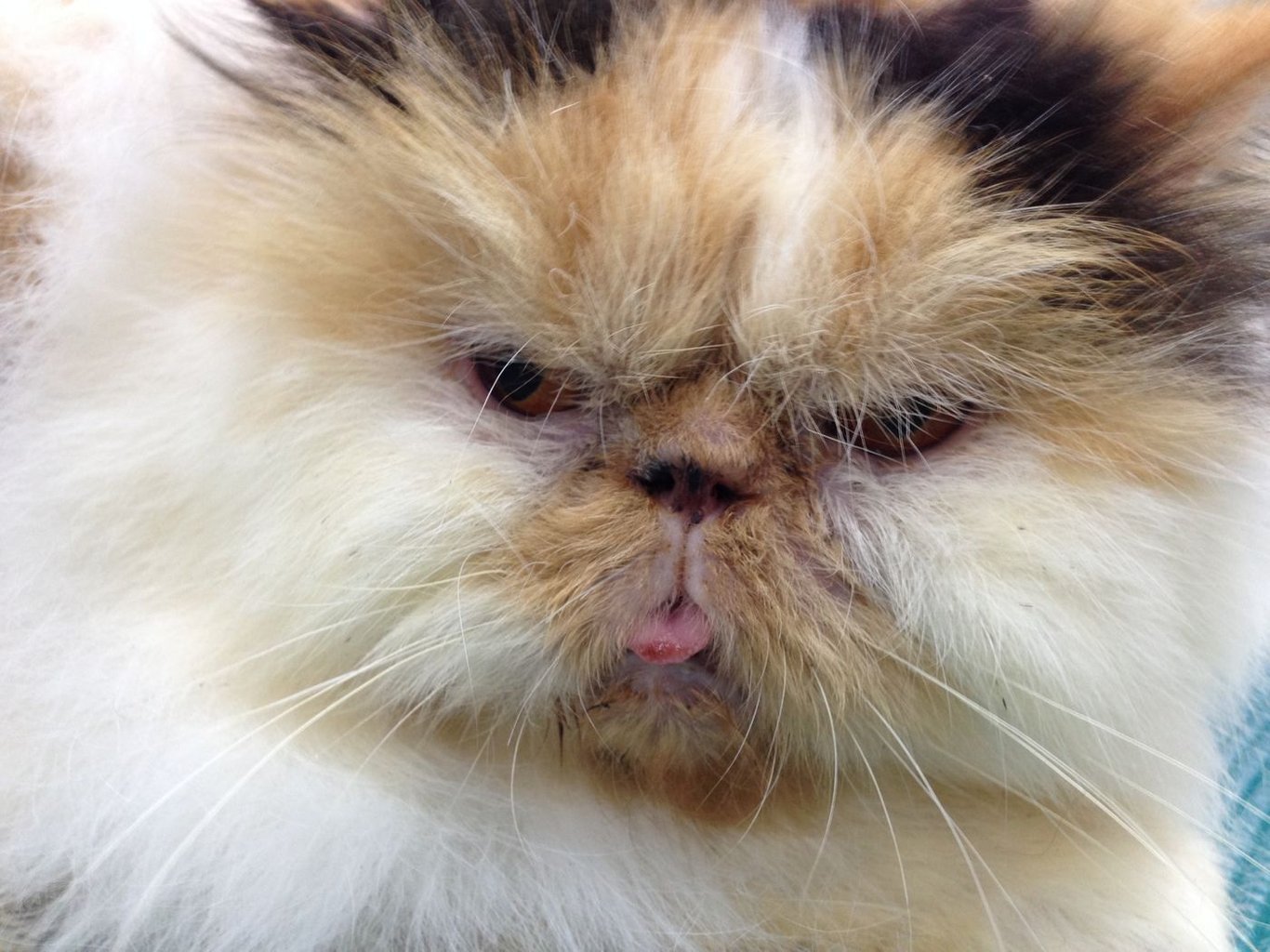
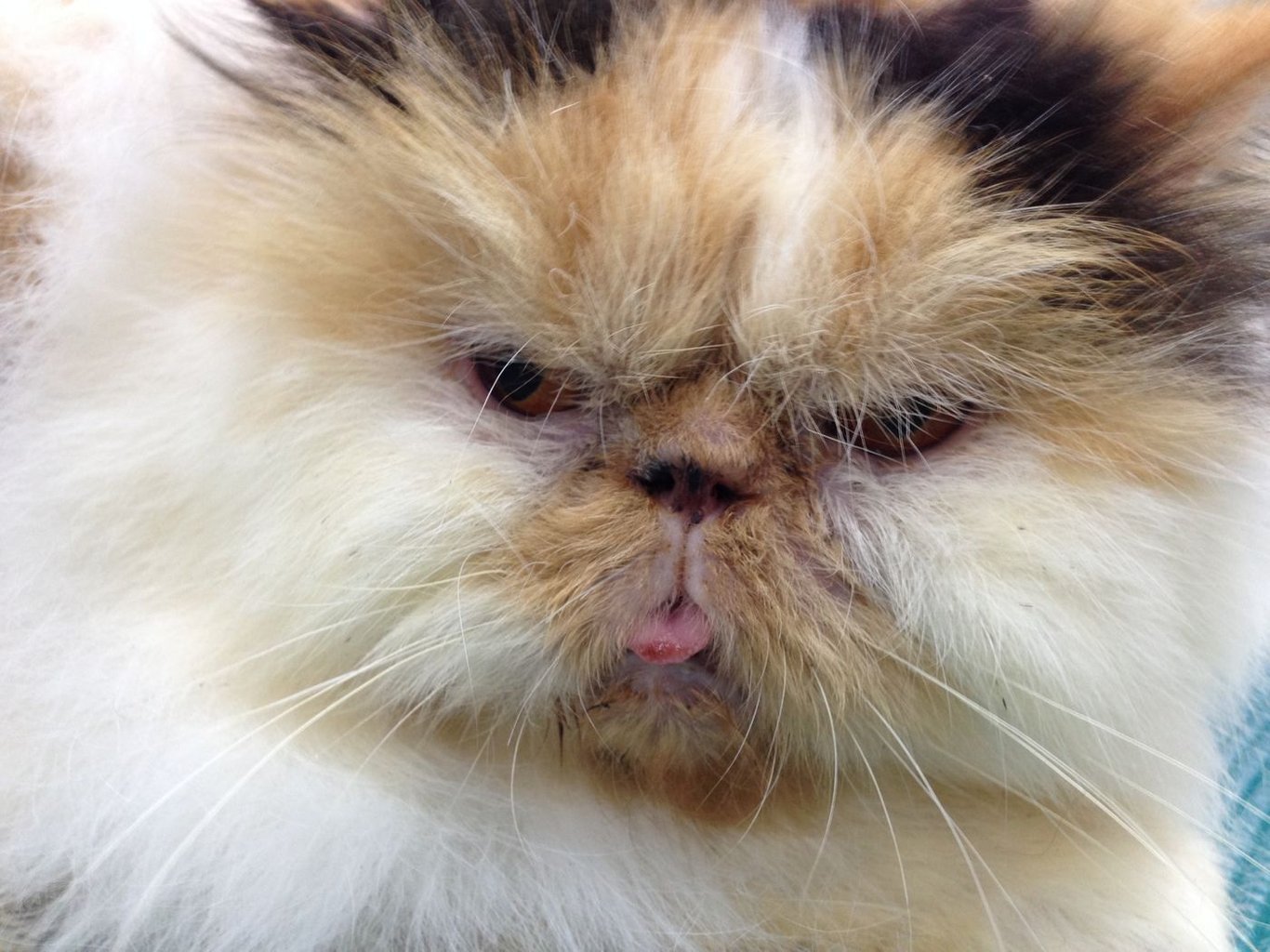
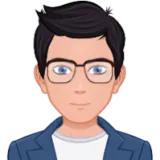
- Author
- More