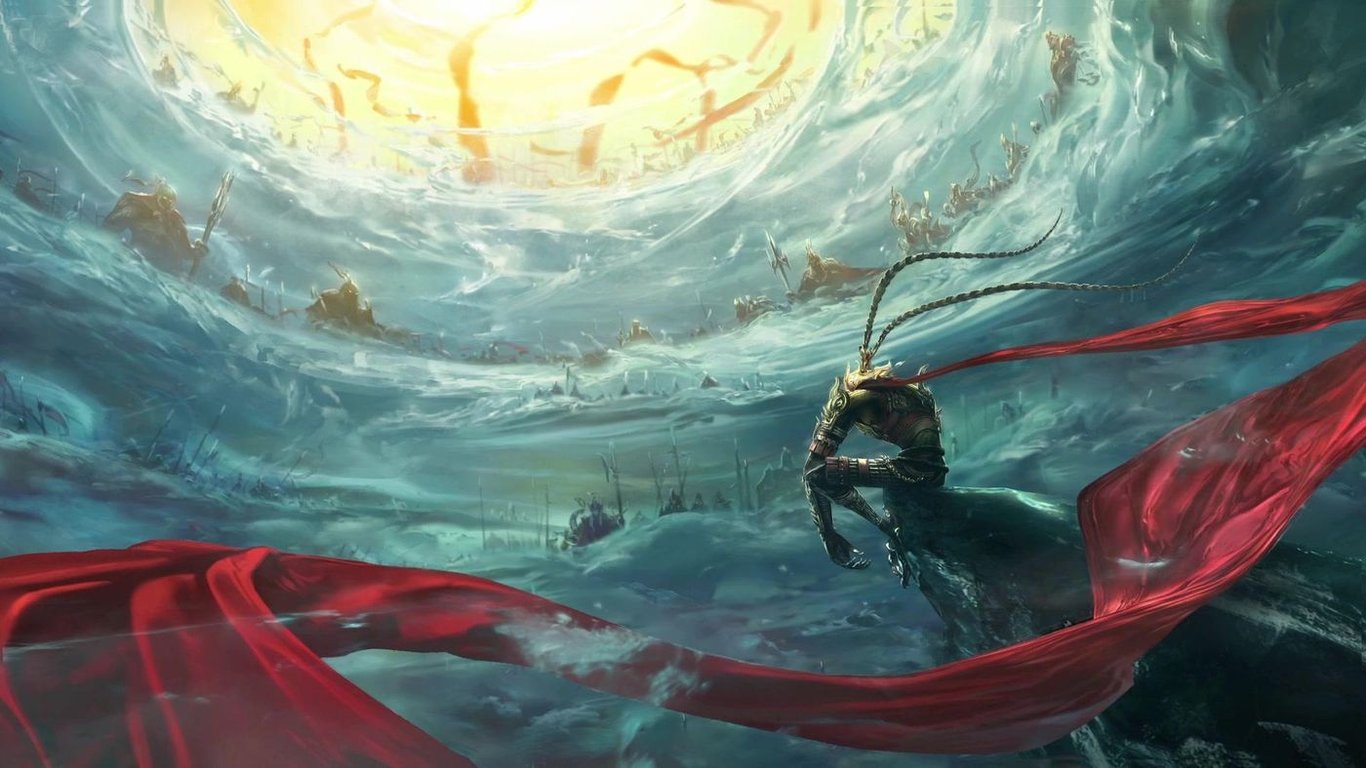
好讀書,修心理學的打工人。
Python Study Notes 1: Basics
Python Study Notes 1: Basics
Python interpreter
Skill 1
When turning the drive letter, you don't need to enter cd, and only use cd to jump to the folder within the drive letter. I put the .py file on the e drive. After the .py file is built, I must first enter e: to jump to the e drive, and then use the cd e:\ folder name to jump to the folder.
For example: when directly transferring from C drive to E drive, directly input:
E:
Navigate from the E drive to the folder under the E drive, and enter:
cd: E:\folder name\folder name
python string
Several functions:
-
ord()
- Get the integer representation of a character
-
chr()
- Convert the encoding to the corresponding character
- Python uses single or double quotes prefixed with b to represent data of type bytes
-
encode()
- coding
-
decode()
- decoding
-
\x##
- Cannot display as ASCII encoding
-
len()
- count the number of characters in
str
- Two line comments:
#!usr/bin/env python3 # _*_coding: utf-8 _*_
%
represents a placeholder, among which%d
integer,%f
float,%s
string,%x
hexadecimal integer.%s
will convert any data type to string,format()
uses the incoming parameters in turn Replace the placeholders{0}
,{1}
, etc. in the string. The difference betweenf-string
and ordinary strings is that if the string contains{xxx}
, it will be replaced with the corresponding variable
# placeholder example >>> 'Hello, %s' % 'world' 'Hello, world' # String placeholder and integer placeholder example >>> 'Hi, %s, you have $%d.' % ('Michael', 1000000) 'Hi, Michael, you have $1000000.' # Format integers and floating-point numbers, such as: whether to add 0, define the number of decimal places >>> print('%2d-%02d' % (3, 1)) >>> print('%.2f' % 3.1415926) 3-01 3.14 #Use s% placeholder >>> 'Age: %s. Gender: %s' % (25, True) 'Age: 25. Gender: True' # Use %% escape characters >>> 'growth rate: %d %%' % 7 'growth rate: 7%' # Use format() to format >>> 'Hello, {0}, the score improved by {1:.1f}%'.format('Xiao Ming', 17.125) 'Hello, Xiao Ming, your grades have improved by 17.1%' # Format with f-string >>> r = 2.5 >>> s = 3.14*r**2 >>> print(f'The area of a circle with radius {r} is {s:.2f}') The area of a circle with radius 2.5 is 19.62
Lists and tuples
- list is an ordered set, elements can be added or deleted, tuple is an ordered set, and elements cannot be added or deleted at will. Among them, each list and tuple can contain other lists or tuples
-
list. append('xxx')
, appends the element 'xxx' to the end -
list [0], list[1]
. Access the element at index position 1,2. Element index in Python starts from 0, that is, index position 0 represents the first element -
list[-1]
, access the last element -
pop(i)
, delete the element whose index position is i, pop() means delete the last element -
list[i]= 'XYZ'
, assign XYZ to the element at index position i, that is, replace the element at index position i with 'XYZ'
>>> s = ['python', 'java', ['asp', 'php'], 'scheme']
- To get
'php'
writep[1]
ors[2][1]
- To define a tuple with only one element, a comma must be added
,
>>> t = (1,) >>> t (1,)
Conditional judgment
if()
else()
elif()
executes conditional judgment, judges from top to bottom and outputs the result, for example:
age = 20 if age >= 6: print('teenager') elif age >= 18: print('adult') else: print('kid')
Note: The colon at the end of the conditional judgment statement :
cannot be dropped
In addition, the error example in the conditional judgment, in this example, pay attention to the first two lines of the code:
s = input('birth: ') birth = int(s) if birth < 2000: print('before 00') else: print('after 00')
If the second line is omitted, an error will be reported when the year is entered directly, because the data type returned by input()
is str
, and str
cannot be directly compared with an integer, and str
must be converted to an integer first. Python provides the int()
function to do this. Therefore, str
should be converted to int
or other variable types.
loop statement
- The
for x in ...
loop is to substitute each element into the variablex
, and then execute the statement of the indented block
L = ['Bart', 'Lisa', 'Adam'] for name in L: print ('Hello,',name,'!')
The result of running the above code is:
Hello, Bart! Hello, Lisa! Hello, Adam!
It should be noted that in the last line of the print statement, in order to output the above results, a comma must be added between different variables. Otherwise ,
the name
cannot be recognized as a variable. That is to say, there must be a distinction between variables and strings. Otherwise, it will not be recognized by the computer.
In the print('Hello,' , name, '!')
statement, name
is a variable not str, so name is written as (name)
without parentheses.
- Python provides a
range()
function that can generate a sequence of integers, which can then be converted to a list by thelist()
function. For example , the sequence generated byrange(5)
is an integer starting from 0 and less than 5:
>>> list(range(5)) [0, 1, 2, 3, 4]
Note: Indentation between code blocks
- The while loop keeps looping as long as the conditions are met, and exits the loop when the conditions are not met.
n = 0 while n < 101: n = n +1, print(n)
- break statement: abort the loop
n = 1 while n <= 100: if n > 10: # When n = 11, the condition is satisfied and the break statement is executed break # The break statement will end the current loop print(n) n = n + 1 print('END')
- continue statement: end the current loop in advance and start the next loop directly.
n = 0 while n < 10: n = n + 1 if n % 2 == 0: # If n is an even number, execute the continue statement continue # The continue statement will directly continue the next cycle, and the subsequent print() statement will not execute print(n)
summary
Loops are an efficient way to get a computer to do repetitive tasks.
The break
statement can directly exit the loop during the loop, and the continue
statement can end the current loop in advance and directly start the next loop. Both of these statements must usually be used in conjunction with the if
statement.
Take special care not to abuse break
and continue
statements. break
and continue
will cause too many logical forks of code execution, which are prone to errors.
Sometimes, if the code is written incorrectly, it will cause the program to fall into an "infinite loop", that is, to loop forever. At this point, you can use Ctrl+C
to exit the program, or force the end of the Python process.
using dict and set
Python has a built-in dictionary: the support of dict, the full name of dict is dictionary, also called map in other languages, uses key-value (key-value) storage, and has extremely fast search speed.
Similar to dict, set is also a collection of keys, but does not store value. Since keys cannot be repeated, there are no repeated keys in the set. To create a set, provide a list as the input set.
- dict
>>> d = {'Michael': 95, 'Bob': 75, 'Tracy': 85} >>> d['Michael'] 95 >>> 'Thomas' in d #Determine whether a key is in dict False >>> d.get('Thomas') #Get a certain key, if there is no such key, return none (note: null values are not displayed in python) >>> d.get('Thomas', -1) #Get a key, if there is no such key, return the specified value -1 >>> d.pop('Bob') #Delete a key and its corresponding value 75 >>> d {'Michael': 95, 'Tracy': 85}
- set
>>> s = set([1, 2, 3]) #define a set >>> s {1, 2, 3} >>> s = set([1, 1, 2, 2, 3, 3]) >>> s {1, 2, 3} #The return value is not repeated >>> s.add(4) #Add a key to the set >>> s {1, 2, 3, 4} >>> s.remove(4) #Remove a key from the set >>> s {1, 2, 3} >>> s1 = set([1, 2, 3]) >>> s2 = set([2, 3, 4]) >>> s1 & s2 #Seek the intersection of two sets{2, 3} >>> s1 | s2 #Seek the union of two sets {1, 2, 3, 4}
About Hash Tables
To understand the relevant content of dict, you need to understand the basic knowledge of hash table (map), which is actually the content in "Algorithms and Data Structures".
1. List and tuple are actually stored in linked list order, that is, the position of the next element is stored in the previous element, so that as long as the position of the first element is found, the position of all the elements can be found along the way, so the name of the list is actually It is a pointer to the position of the first element of the list. The insertion and deletion of lists can be done directly in the form of linked lists. For example, if I want to insert an element between the first element and the second element, then directly at the end of the linked list (we assume that this list has only two elements, then That is, at the position of the third element) insert this element, then point the first element pointer to this element (the third position), and then point the pointer of the newly inserted element to the original second element, so The insert operation is complete. When reading this list, first use the name of the list (that is, a pointer to the position of the first element) to find the first element, then use the pointer of the first element to find the second element (position 3), and then Find the 3rd element (position 2) with the pointer of the 2nd element, and so on. So the order of the list does not necessarily correspond exactly to the actual order in memory. This storage method does not waste memory, but it takes a lot of time to search, because you have to search one by one according to the linked list. If your list is very large, it will take a long time to find the result.
2.dict uses a special method, a hash table, for fast lookup. The hash table uses a hash function to calculate a number from the key (the hash function has a characteristic: for different keys, there is a high probability that the obtained hash value will be different), and then directly store the value in the corresponding number. address, such as key='ABC', value=10, the hash value corresponding to the key obtained through the hash function is 123, then apply for a memory with 1000 addresses (from 0 to 999), and then store 10 at address 123. Similarly, for key='BCD', value=20, and the hash value of the key is 234, then store 20 at the address of 234. It is very convenient to look up such a table. Just give the key, calculate the hash value, and then go directly to the corresponding address to find the value. No matter how many elements there are, the value can be found directly without traversing the entire table. However, although the dict search speed is fast, the memory waste is serious. You see that we only store two elements, and we have to apply for a memory with a length of 1000.
3. Now you know why the key uses an immutable object, right? Because immutable objects are constants, each hash value is fixed, so there is no error. For example, key='ABC', value=10, and the storage address is 123. Suppose I change the key to 'BCD' on a whim, then when looking for the value of 'BCD', I will go to the address of 234, but there Nothing, it's a mess.
3. You see we have a sentence above: for different keys, there is a high probability that the hash values obtained are also different. So there is a small probability that different keys can get the same hash value? That's right, for example, for our example, the hash value is only 3 bits, so as long as the number of elements exceeds 1000, there must be at least two keys with the same hash value (pigeon cage principle), this situation is called " Conflicts", when designing hash tables, we must take measures to reduce conflicts, and we must find ways to remedy the conflicts. However, this is a matter of the compiler, and for beginners, the probability of encountering conflicts is basically equal to zero, so there is no need to worry about it.
Like my work?
Don't forget to support or like, so I know you are with me..
Comment…