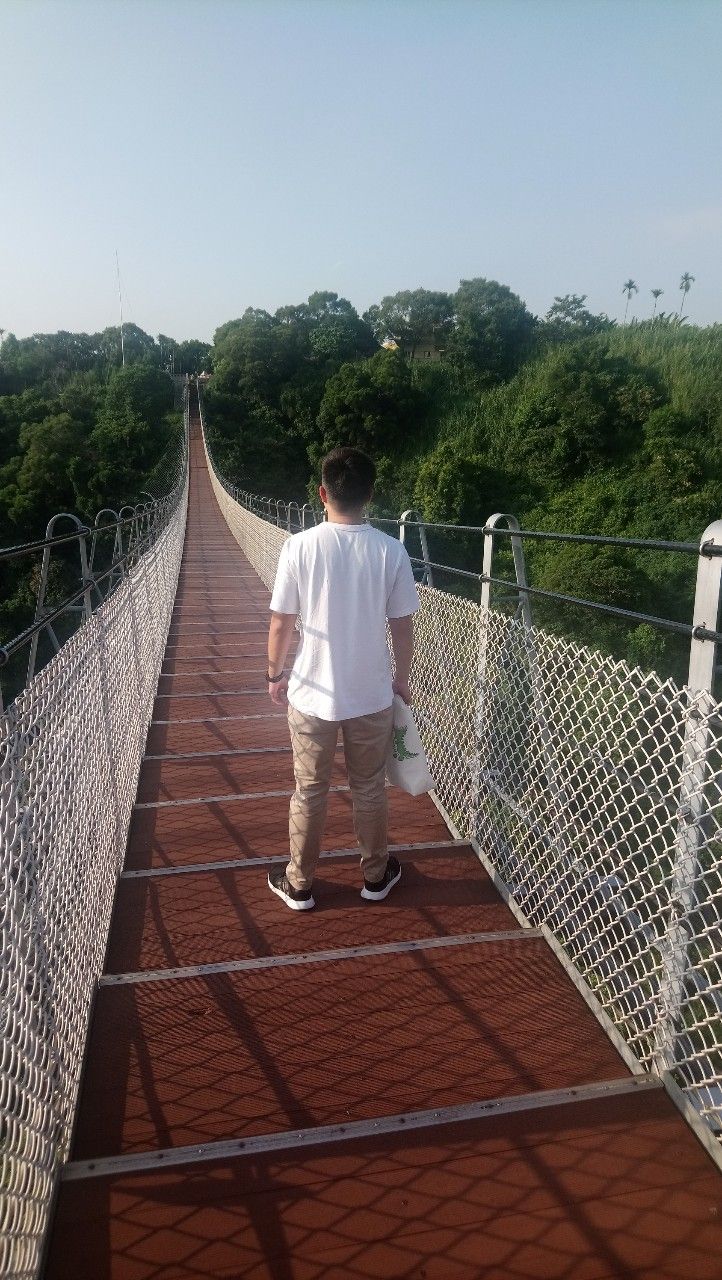
YO~~ 剛跨入AI人工智慧領域的小小工程師, 熱愛自學, 熱愛分享, 下班後的我想為自己Coding, 積極撰寫教學文, 想將自學的程式知識分享給大家, 不斷追求進步的自己, 希望有一天能回饋社會,幫助需要幫助的人, 如果您有什麼很酷的想法,也覺得我還行,歡迎您找我合作~~ IG: https://www.instagram.com/coding_4_me/
LeetCode Study Notes - How to Start Using LeetCode to Brush Questions - First Experience of Brushing LeetCode - LeetCode Question 1 - Two Sum Solution
Github link
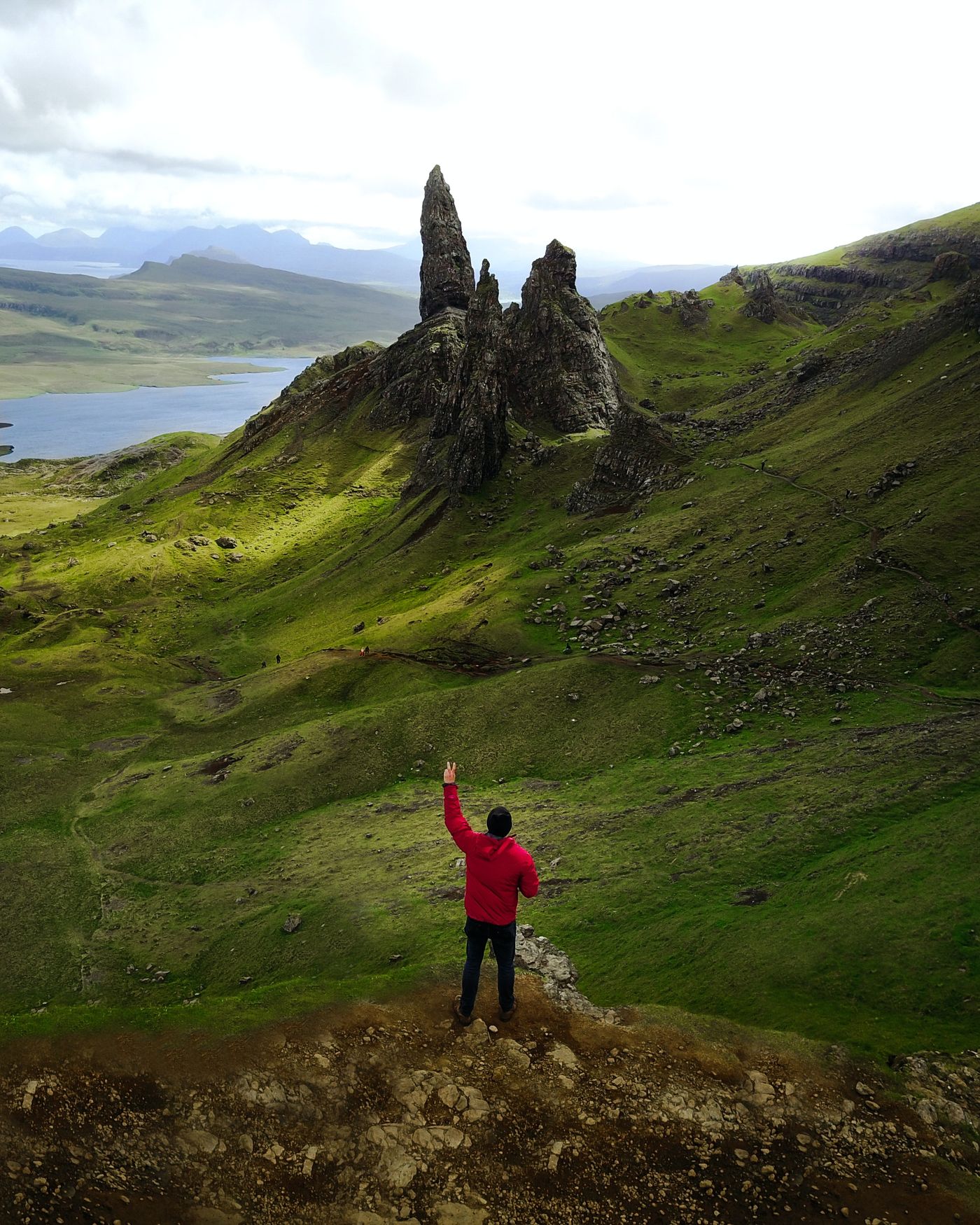
1. How to get started on the LeetCode website?
Step 1: Open the LeetCode website and log in
Step 2: Click on Problems
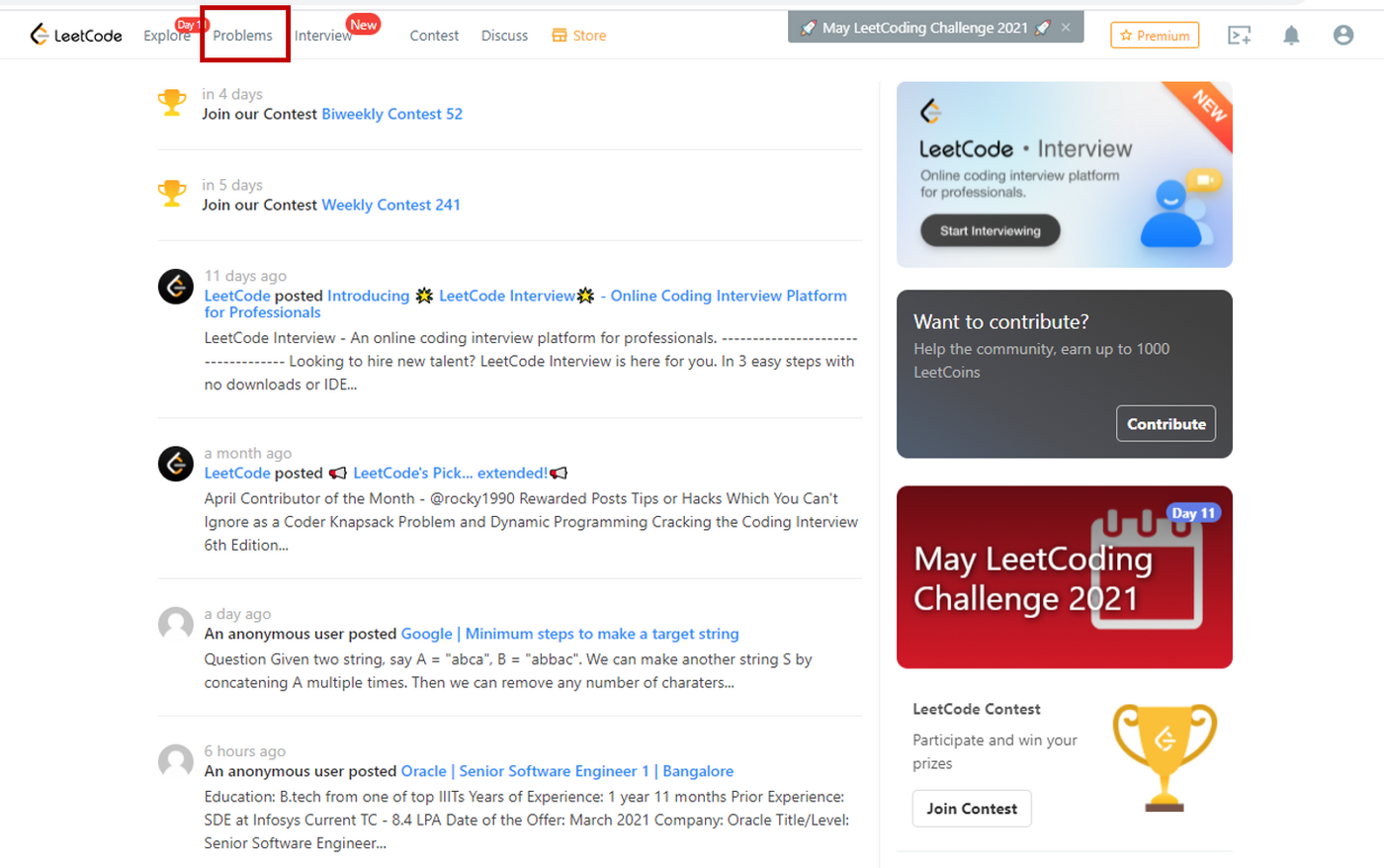
Step 3: Fill in the topics and choose the category topics you want
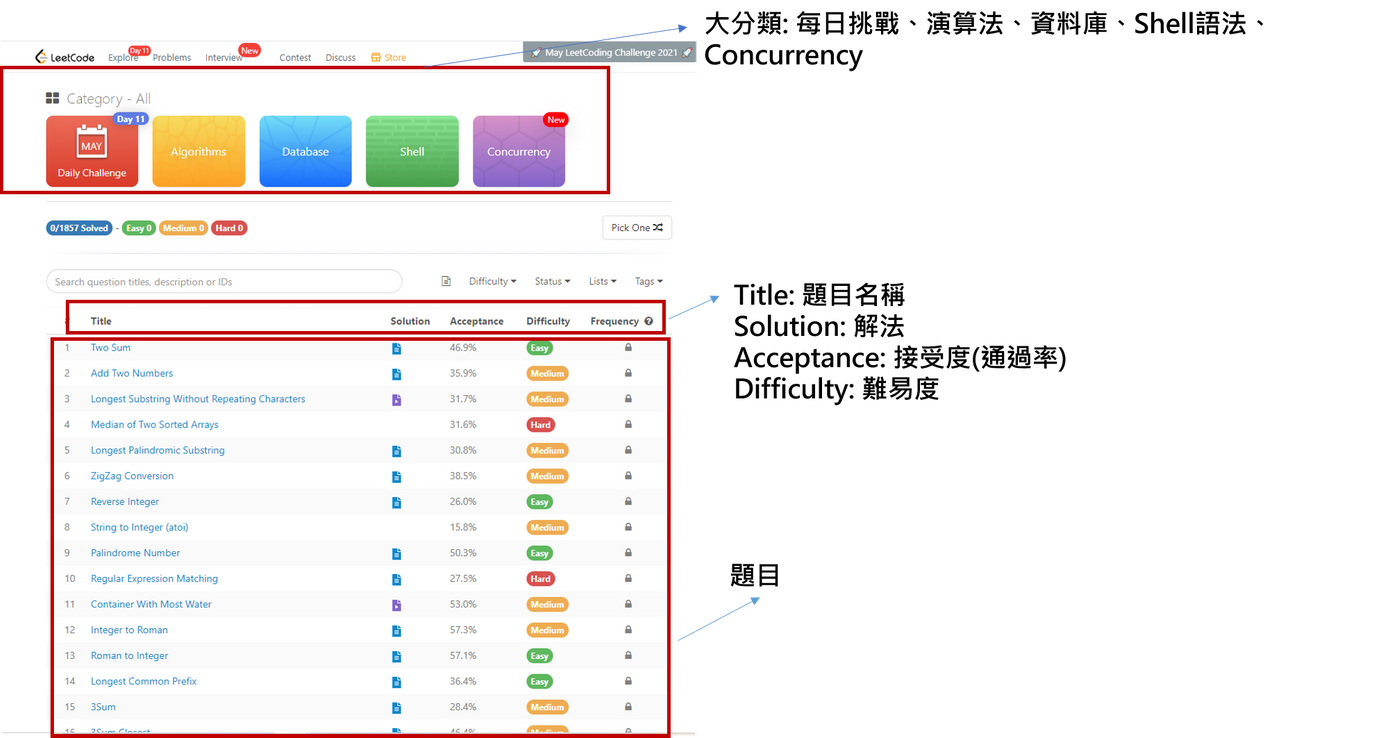
Step 4: Select a good question, click in, and you can start to brush the question (Two Sum as an example)
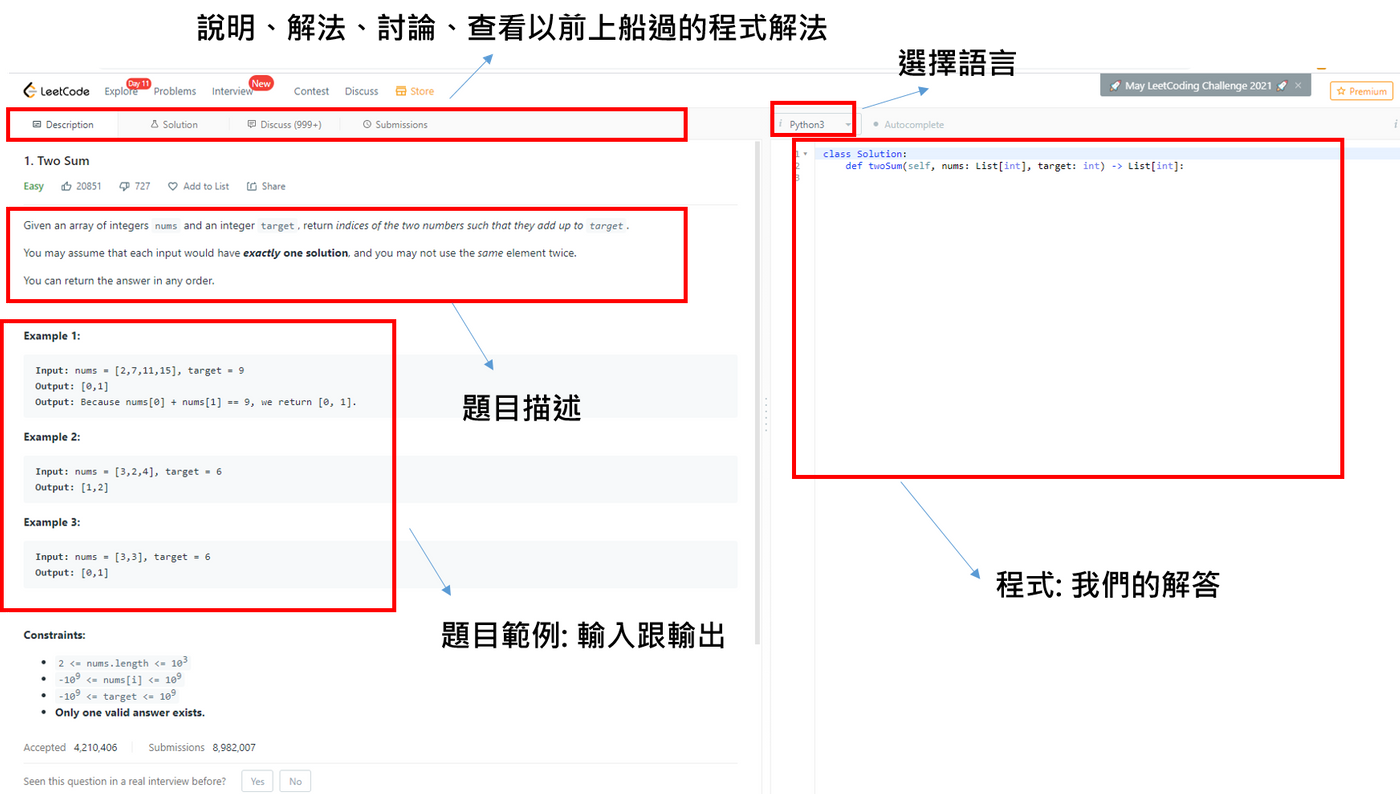
2. Two Sum implementation
topic:
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
You may assume that each input would have *exactly* one solution , and you may not use the same element twice.
You can return the answer in any order.
Given a nums array of integers and a target integer, the program will return the indices of the two numbers in the array, that is, the two numbers will add up to the target integer
You can assume that there is only one solution per input and the same element will not be reused, the answer can be returned in any order
Solution 1: Violent solution
Description: First take a fixed element from the list, and then start pairing one by one from its next element to see if the addition is equal to the target value, if not, replace the next element, and so on
def two_sum(nums, target): for i in range(len(nums)): for j in range(i+1, len(nums)): if nums[i] + nums[j] == target: return i, j nums = [1, 2, 3, 4, 5, 6] target = 10 two_sum(nums, target)
Results of the
(3, 5)
Reminder: Although the violent solution is easy to understand, it is a very bad choice for the program execution performance. Unless it is not possible, I hope everyone can use the violent solution less.
Solution 2: Hash Table Method - Hash Method
Description: Create a dictionary to load the value and the index, the value is placed in the key, and the index is placed in the value. When the program starts to execute, because no data has been added to the previous hash_table, it must be empty, and then the data in front of nums is slowly filled. After entering, to the next element, hash_table also has a lot of data, it may be added to the previous element to equal the target value, and then it can be returned
method one
class Solution: def two_sum(self, nums, target): hash_table = {} for i, num in enumerate(nums): t = target - num if t in hash_table: return [hash_table[t], i] ## Add data to hash table (key: value, value: index) hash_table[num] = i ## Return [-1, -1] when no answer is found return [-1, -1] # print(hash_table) nums = [1, 2, 3, 4, 5, 6] target = 10 Solution().two_sum(nums, target)
Results of the
[3, 5]
Method Two
class Solution: def two_sum(self, nums, target): hash_map = {} for i in range(len(nums)): if target - nums[i] in hash_map: return hash_map.get(target - nums[i]), i hash_map[nums[i]] = i nums = [1, 2, 3, 4, 5, 6] target = 10 Solution().two_sum(nums, target)
I tested the execution time on LeetCode, the method will be slightly faster!!
Like my work?
Don't forget to support or like, so I know you are with me..
Comment…